- Denisse's Newsletter
- Posts
- 🧠 If you only solve one problem this week, let it be this!
🧠 If you only solve one problem this week, let it be this!
Day 4 : 103. Binary Tree Zigzag Level Order Traversal

Try the internet’s easiest File API
Tired of spending hours setting up file management systems? Pinata’s File API makes it effortless. With simple integration, you can add file uploads and retrieval to your app in minutes, allowing you to focus on building features instead of wasting time on unnecessary configurations. Our API provides fast, secure, and scalable file management without the hassle of maintaining infrastructure.
TLDR
Welcome!
Zig Zag Level Order
Recommendations I live by!
Self-Gaslight of the week
JOBS & SCHOLARSHIPS
Hello and welcome back brave human being!
If you've been paying attention, you're well aware of Sabrina Carpenter, Chappell Roan, and Charli XCX. To the casual observer, it might seem like these artists shot to fame overnight.
But my brave human being, it is quite the contrary.
Each of them spent over a decade honing their craft before finally breaking through in 2024.
It takes 10 years to build a career—10 years of relentless effort, showing up day in and day out.
So let’s put in the work today and start building what’s to come!
LFG!!
LeetCode problem
Given the root of a binary tree, return the bottom-up level order traversal of its nodes' values. (i.e., from left to right, level by level from leaf to root).
Before we dive in,
Breathe
Nothing is ever that deep.
Now, let’s
But there is a little trick that I am about to teach you….
We’re going to leverage recursion to navigate through the tree and a map to store the nodes level by level, where the key is the level and the value is an array with the nodes in that level.
We are simply going to iterate through the tree INORDER, and store in our map the current node we are processing and their level:
treeNodes[level].push_back(root->val);
And every time we call our recursive function, we’re simply going to add 1 to our level so that when we traverse deeper into the tree, we know exactly where we are:
auxLevelOrder(root->left, level + 1);
auxLevelOrder(root->right, level + 1);
This approach allows us to group nodes together INORDER by their levels as we go down the tree.
Once we build our map of levels and their respective nodes, we iterate through the map IN REVERSE!!!!
Step by Step solution!
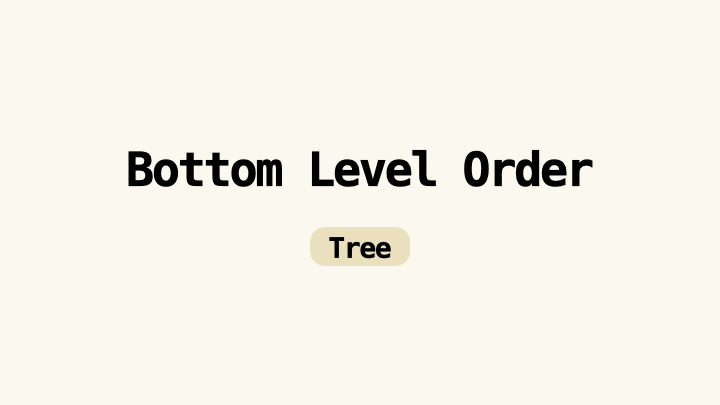
Complete Solution :
class Solution {
public:
map<int, vector<int>> LeveNodes; // key: level, value: nodes of level
void InOrder(TreeNode* root, int level) {
if (root != nullptr) {
InOrder(root->left, level + 1);
LeveNodes[level].push_back(root->val);
InOrder(root->right, level + 1);
}
}
vector<vector<int>> levelOrderBottom(TreeNode* root) {
InOrder(root, 0);
vector<vector<int>> result;
// loop through the reverse map and push_back
for (auto it = LeveNodes.rbegin(); it != LeveNodes.rend(); ++it) {
result.push_back(it->second);
}
return result;
}
};
Recommendations
Level up your inbox by subscribing to what I am subscribed to!
Hacks of the week
Controversial!! “Fake” a forwarded email to someone asking for an introduction 👀
Self-gaslight of the week
I truly believe you can gaslight yourself to success.
Write a letter to your future self, remembering all the fights you had to get where you are
Create something to look forward to tomorrow, for me its coffee!!!
Jobs and Scholarships
Cheers to you hacking your week,
Denisse
Writer RAG tool: build production-ready RAG apps in minutes
RAG in just a few lines of code? We’ve launched a predefined RAG tool on our developer platform, making it easy to bring your data into a Knowledge Graph and interact with it with AI. With a single API call, writer LLMs will intelligently call the RAG tool to chat with your data.
Integrated into Writer’s full-stack platform, it eliminates the need for complex vendor RAG setups, making it quick to build scalable, highly accurate AI workflows just by passing a graph ID of your data as a parameter to your RAG tool.
Reply